Sorting
Sorting can be managed through the sort
prop of the MuuriComponent. The sort will be updated every time that the MuuriComponent re-render
with a new value in the prop or an Item is added.
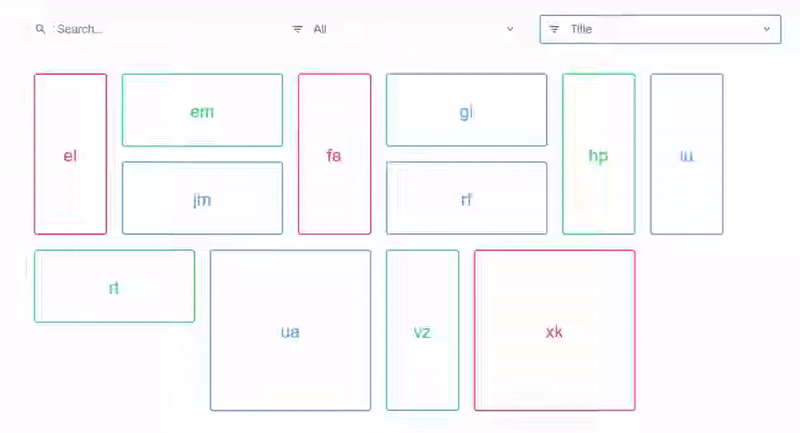
The MuuriComponent sorts the DOM elements
according to how it is set up, this sorting has nothing to do with the sorting of the Item
components you use. In fact, you will never have to sort the Item
components, they can stay (or be added) in any position.
3 ways โ๏ธ
There are two ways to sort the Items based on their data:
- Using a
function
as the comparer, which will receive the data of two Items to compare as arguments. It works almost identically to native array sort, the only difference is that the sort is always stable. - Using the data key(s) as a
string
(separated by space) and the Items will be sorted based on the provided data keys.
There is one other way that is not based on data:
- Using an ordered list of Items
keys
.
Usage ๐ (function)
In the example the Items are distinguished by an id
, we want to sort them on that id.
Usage ๐ (string)
In the example the Items are distinguished by an id and a text
, we want to sort them on that text. If 2 Items have the same text, we will sort them by their id
(in descending
order).
Usage ๐ (keys)
In the example the Items will be sorted by their keys
.
Sort options โ๏ธ
You can decide to sort in descending
order using the sortOptions. If you want to avoid unnecessary re-sorting during a re-render, remember to memoize the options.
Store sort order ๐พ
You can get the ordered array of keys of the Item
components. This array can be used at any time to sort the Items.
It is possible to store the keys after a drag event ๐พ.
It is also possible to store them after each new sort ๐พ.
And then apply the sort ๐.
As said before you don't have to worry about the position of the components, they can be in any order.
How is that possible? ๐คจ
You may have wondered how the sorting of the Item
components does not affect the sorting of DOM elements. This is because the elements are positioned via CSS properties, so you don't have to reorder the Item
components after the sort is applied. This allows an extremely simple use of the library.
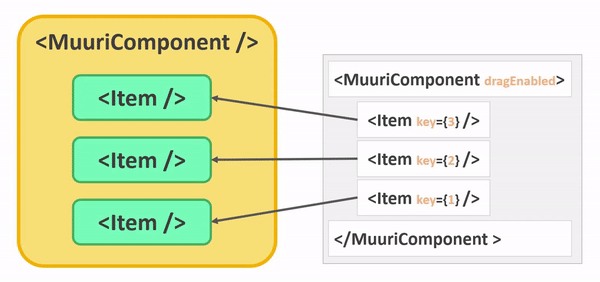